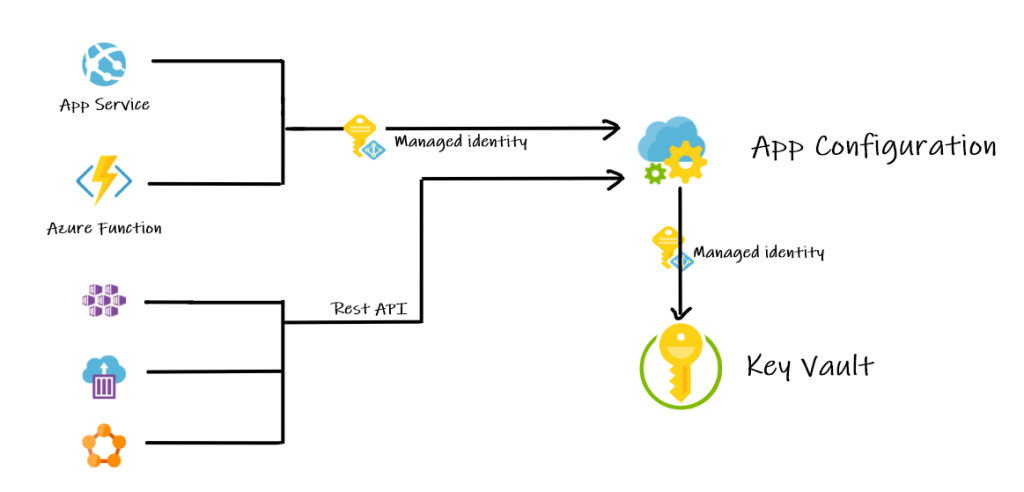
NOTE: This article assumes you have a good handle on Azure-managed Identity and Key Vault. If not, links to more information can be found throughout the article.
What is Azure App Configuration?
Software products store application configuration either on the code itself or on external configuration files. Modern, cloud-based applications rely on substantially more configuration data than traditional applications do, and this configuration is usually deployed to multiple environments. If you have worked on smaller services, you may have copied the same configuration onto multiple services, which makes maintaining them much more challenging.
Azure App Configuration provides an easy way out. This innovative, Azure-managed service helps developers to centrally maintain application settings and feature flags. All distinct components, along with all settings, are stored in a single, secure place. Simply put, Azure App Configuration has been designed to help developers optimise their work, be more secure, and avoid duplication or out of synch. data which makes them more productive end-to-end.
Why Azure App Configuration?
We have established that developers need a centralized and robustly secure platform for managing and scaling cloud-based applications efficiently. Azure App Configuration serves this purpose. Although almost all cloud-based applications can benefit from the App Configuration, some can maximise the advantages which the service provides as follows:
- Serverless apps based on event-driven, stateless compute apps or other Azure functions.
- Microservices apps that include containerized apps such as Azure Kubernetes Service, Azure Service Fabric, and deployed in one or more geographical zones.
The benefits offered by the App Configuration include:
- set up in a few minutes and provides point-in-time replay of settings
- built-in support for Azure managed identities to help your website
- flexible key mappings and representations
- increased code security de through Azure-managed identities
- complements Azure key vault and simplifies real-time control of the availability of all features
- quick and efficient integration with other well-known frameworks.
- strong security or encryption of sensitive data, both at rest and in transit
- compares two different sets of configurations based on custom-defined dimensions
- user interface for feature flag management
Many more features are available; read about them here https://docs.microsoft.com/en-us/azure/azure-app-configuration/overview
App Configuration integration with Key Vault
Modern applications consist of secrets, keys, and configuration. Even though Azure App Configuration can keep secrets and keys, App Configuration is not designed to do this. Therefore, we need a combination of Azure App Configuration and Key Vault.
This article demonstrates how you can take advantage of Azure App Configuration with Azure-managed Identity and Key Vault.
Prerequisites
- Azure account with a subscription
- Azure CLI
- Coffee
Create an App Configuration store
To create a new App Configuration store, sign in to the azure cli.
az login
If you have multiple subscriptions, please select the subscription.
az account set --subscription “subscription name”
Let’s create App Configuration and resource group
az group create --name SotronConfigStore --location NorthEurope
az appconfig create --name TestAppConfigStore --location NorthEurope --resource-group SotronConfigStore --sku Free
Enable system-assigned identity for this configuration store
az appconfig identity assign --name TestAppConfigStore --resource-group SotronConfigStore
Create Key Vault
az keyvault create --location NorthEurope --name SotronKeyStore --resource-group SotronConfigStore
Add a secret to Key Vault
az keyvault secret set --vault-name SotronKeyStore -n welcomeMessage --value "Hello from KeyVault"
Allow App configuration to access secrets.
Before you run the following script, replace {object-id} with TestAppConfigStore object Id
az keyvault set-policy --name SotronKeyStore --object-id {object-id} --secret-permissions get
Create App Setting referencing Key Vault secret
Let us add configuration which references the Key Vault secret we have created. Please replace the identifier with your identifier.
az appconfig kv set-keyvault -n TestAppConfigStore --key welcomeMessage --label dev --secret-identifier https://sotronkeystore.vault.azure.net/secrets/welcomeMessage/4cfd3d38c8924436a8af205c94839a13
Access App Configuration using .NET application
Github repo: https://github.com/nishanperera/Azure-App-Configuration-With-Key-Vault
Create a default an ASP.Net Core Web Application and install “Azure.Identity” package make sure to install the preview version.
Install-Package Azure.Identity -Version 1.2.0-preview.4
Add the following to appsetting.json
"AppConfig": {
"Endpoint": "https://{yourAppConfigurationEndpoint}.azconfig.io"
}
Replace CreateHostBuilder with following.
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
webBuilder.ConfigureAppConfiguration((hostingContext, config) =>
{
var settings = config.Build();
var credentials = new DefaultAzureCredential(
new DefaultAzureCredentialOptions
{
ExcludeVisualStudioCredential = true
});
config.AddAzureAppConfiguration(options =>
{
options.Connect(new Uri(settings["AppConfig:Endpoint"]), credentials)
.ConfigureKeyVault(kv =>
{
kv.SetCredential(credentials);
})
.Select(KeyFilter.Any, "dev");
});
}).UseStartup<Startup>());
Now you can access the configuration using:
Configuration["welcomeMessage"]
To run the code locally
Grant the “App Configuration Data Reader” role to your user account.
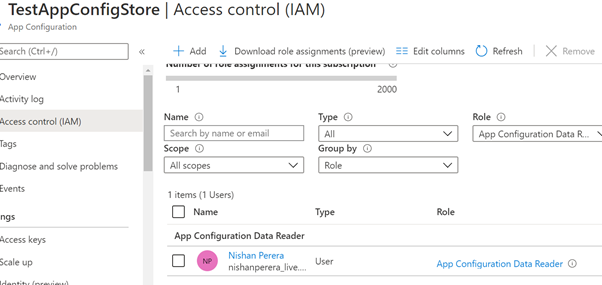
Next, grant access to Key Vault.
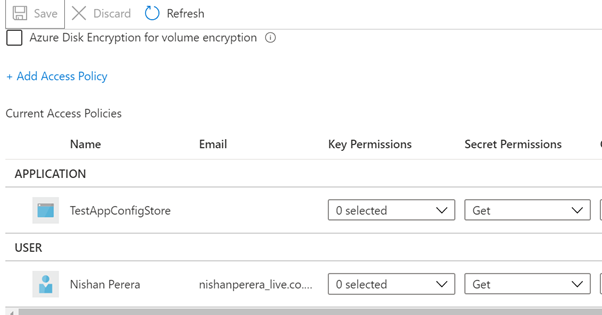